Terraform IAM Management
Introduction
In today’s competitive cloud computing landscape, effectively managing AWS Identity and Access Management (IAM) users has become crucial for businesses. By leveraging Terraform IAM, you can automate and streamline the management process for your AWS IAM users, ensuring a secure and well-organized infrastructure. In this blog post, we’ll guide you through managing AWS IAM users with Terraform by providing a step-by-step explanation and examples.
Understanding AWS IAM and Terraform
Before diving into managing AWS IAM users with Terraform, it’s essential to understand the basics of AWS IAM and Terraform.
AWS IAM: AWS Identity and Access Management (IAM) is a web service that allows you to control access to AWS resources securely. You can create and manage users, groups, and permissions, ensuring that only authorized personnel have access to your cloud resources.
Terraform is an open-source infrastructure as code (IaC) tool that enables you to define, provision, and manage cloud resources using a declarative language called HashiCorp Configuration Language (HCL). Terraform is cloud-agnostic and supports multiple providers, including AWS.
Getting Started with Terraform IAM
To begin managing AWS IAM users with Terraform, you’ll need to set up your environment.
Prerequisites:
- AWS account
- Terraform installed
- AWS CLI installed and configured
Initialize Terraform: Create a new directory for your Terraform project and navigate to it. Then, create a main.tf
file with the following content:
provider "aws" {
region = "us-west-2"
}
Run terraform init
to initialize your Terraform workspace and download the AWS provider.
Creating and Managing AWS IAM Users with Terraform
Now that your environment is set up, let’s dive into managing AWS IAM users with Terraform.
Creating an IAM User:
In your main.tf
file, add the following Terraform configuration to create an IAM user:
resource "aws_iam_user" "example_user" {
name = "example-user"
}
Run terraform apply
to create the IAM user. Confirm the changes by typing yes
when prompted.
Creating an IAM Group:
Add the following Terraform configuration to your main.tf
file to create an IAM group:
resource "aws_iam_group" "example_group" {
name = "example-group"
}
Run terraform apply
to create the IAM group.
Attaching an IAM Policy to the Group:
Add the following Terraform configuration to your main.tf
file to attach a managed IAM policy to the group:
resource "aws_iam_group_policy_attachment" "example_group_policy" {
group = aws_iam_group.example_group.name
policy_arn = "arn:aws:iam::aws:policy/AmazonS3ReadOnlyAccess"
}
Run terraform apply
to attach the policy to the group.
Adding the IAM User to the Group:
Add the following Terraform configuration to your main.tf
file to add the IAM user to the group:
resource "aws_iam_group_membership" "example_group_membership" {
name = "example-group-membership"
users = [aws_iam_user.example_user.name]
group = aws_iam_group.example_group.name
}
Creating Multiple IAM Users:
To create multiple IAM users, you can use the count
parameter in your aws_iam_user
resource block. For example, to create three IAM users, update your main.tf
file as follows:
resource "aws_iam_user" "example_users" {
count = 3
name = "example-user-${count.index}"
}
Run terraform apply
to create the three IAM users.
Adding Multiple IAM Users to the Group:
Update the aws_iam_group_membership
block in your main.tf
file to include all the users created in the previous step:
resource "aws_iam_group_membership" "example_group_membership" {
name = "example-group-membership"
users = aws_iam_user.example_users[*].name
group = aws_iam_group.example_group.name
}
Run terraform apply
to add all the IAM users to the group.
Removing an IAM User from the Group:
To remove a specific IAM user from the group, update the aws_iam_group_membership
block in your main.tf
file to exclude the user:
resource "aws_iam_group_membership" "example_group_membership" {
name = "example-group-membership"
users = [
aws_iam_user.example_users[0].name,
aws_iam_user.example_users[2].name,
]
group = aws_iam_group.example_group.name
}
In this example, we have removed the second user (index 1) from the group. Run terraform apply
to apply the changes.
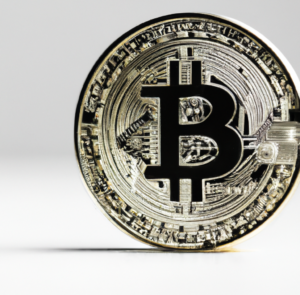
Best Practices for Managing AWS IAM Users with Terraform
When managing AWS and Terraform IAM users, consider these best practices:
Use Terraform IAM Modules: Create reusable Terraform modules to define and manage your IAM users, groups, and policies. This enables you to maintain a consistent and maintainable codebase.
Keep Terraform State Secure: Terraform state files can contain sensitive information. Use remote backends, such as AWS S3, and enable encryption at rest to store your Terraform state files securely.
Version Control: Store your Terraform configuration files in a version control system, such as Git, to track changes, collaborate with your team, and easily revert changes if needed.
By following these best practices, you can ensure a secure, efficient, and well-organized management process for your AWS Terraform IAM users.
Leave a Reply
You must be logged in to post a comment.